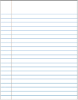
MDTextField
Description 
MDTextField
MDTextField format:
MDTextField: hint_text: 'Persistent helper text' helper_text: 'Text is always here' helper_text_mode: 'persistent' multiline: True
No special library, no append of widget.
Example in code
from kivy.lang import Builder from kivymd.app import MDApp KV = ''' BoxLayout: padding: '10dp' MDTextField: id: text_field_error hint_text: 'Helper text on error (press 'Enter')' helper_text: 'There will always be a mistake' helper_text_mode: 'on_error' pos_hint: {'center_y': .5} ''' class MainApp(MDApp): def __init__(self, **kwargs): super().__init__(**kwargs) self.screen = Builder.load_string(KV) def build(self): self.screen.ids.text_field_error.bind( on_text_validate=self.set_error_message, on_focus=self.set_error_message, ) return self.screen def set_error_message(self, instance_textfield): self.screen.ids.text_field_error.error = True MainApp().run()
ID:(13946, 0)
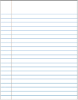
MDTextFieldRect
Description 
MDTextFieldRect
MDTextFieldRect format:
MDTextFieldRect: size_hint: 1, None height: '30dp'
No special library, no append of widget.
Example in code
from kivy.lang import Builder from kivymd.app import MDApp KV = ''' BoxLayout: padding: '10dp' MDTextFieldRect: size_hint: 1, None height: '30dp' pos_hint: {'center_y': .5} ''' class MainApp(MDApp): def __init__(self, **kwargs): super().__init__(**kwargs) self.screen = Builder.load_string(KV) def build(self): self.screen.ids.text_field_error.bind( on_text_validate=self.set_error_message, on_focus=self.set_error_message, ) return self.screen def set_error_message(self, instance_textfield): self.screen.ids.text_field_error.error = True MainApp().run()
ID:(13947, 0)
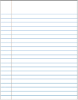
MDTextFieldRound
Description 
MDTextFieldRound
MDTextFieldRound format:
MDTextFieldRound: icon_left: 'key-variant' icon_right: 'eye-off' hint_text: 'Field with left and right icons'
No special library, no append of widget.
Example in code
from kivy.lang import Builder from kivy.properties import StringProperty from kivymd.app import MDApp from kivymd.uix.relativelayout import MDRelativeLayout KV = ''': size_hint_y: None height: text_field.height MDTextFieldRound: id: text_field hint_text: root.hint_text text: root.text password: True color_active: app.theme_cls.primary_light icon_left: 'key-variant' padding: self._lbl_icon_left.texture_size[1] + dp(10) if self.icon_left else dp(15), (self.height / 2) - (self.line_height / 2), self._lbl_icon_right.texture_size[1] + dp(20), 0 MDIconButton: icon: 'eye-off' ripple_scale: .5 pos_hint: {'center_y': .5} pos: text_field.width - self.width + dp(8), 0 on_release: self.icon = 'eye' if self.icon == 'eye-off' else 'eye-off' text_field.password = False if text_field.password is True else True MDScreen: ClickableTextFieldRound: size_hint_x: None width: '300dp' hint_text: 'Password' pos_hint: {'center_x': .5, 'center_y': .5} ''' class ClickableTextFieldRound(MDRelativeLayout): text = StringProperty() hint_text = StringProperty() # Here specify the required parameters for MDTextFieldRound: # [...] class MainApp(MDApp): def build(self): return Builder.load_string(KV) MainApp().run()
ID:(13948, 0)
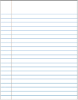
MDCheckbox
Description 
MDCheckbox
MDCheckbox format:
MDCheckbox: size_hint: None, None size: '48dp', '48dp' pos_hint: {'center_x': .5, 'center_y': .5}
No special library, no append of widget.
Example in code
from kivy.lang import Builder from kivymd.app import MDApp KV = ''' MDFloatLayout: MDCheckbox: size_hint: None, None size: '48dp', '48dp' pos_hint: {'center_x': .5, 'center_y': .5} ''' class MainApp(MDApp): def build(self): return Builder.load_string(KV) MainApp().run()
ID:(13949, 0)
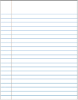
MDCheckbox with Group (Radiobutton)
Description 
MDCheckbox
MDCheckbox format:
: group: 'group' size_hint: None, None size: dp(48), dp(48) MDFloatLayout: Check: active: True pos_hint: {'center_x': .4, 'center_y': .5} Check: pos_hint: {'center_x': .6, 'center_y': .5}
No special library, no append of widget.
Example in code
from kivy.lang import Builder from kivymd.app import MDApp KV = ''': group: 'group' size_hint: None, None size: dp(48), dp(48) MDFloatLayout: Check: active: True pos_hint: {'center_x': .4, 'center_y': .5} Check: pos_hint: {'center_x': .6, 'center_y': .5} ''' class MainApp(MDApp): def build(self): return Builder.load_string(KV) MainApp().run()
ID:(13950, 0)
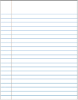
MDSwitch
Description 
MDSwitch
MDSwitch format:
MDSwitch: width: dp(64)
No special library, no append of widget.
Example in code
from kivy.lang import Builder from kivymd.app import MDApp KV = ''' MDFloatLayout: MDSwitch: pos_hint: {'center_x': .5, 'center_y': .5} ''' class MainApp(MDApp): def build(self): return Builder.load_string(KV) MainApp().run()
ID:(13951, 0)
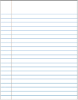
MDDropDownItem
Description 
from kivy.lang import Builder from kivymd.app import MDApp KV = ''' Screen MDDropDownItem: id: drop_item pos_hint: {'center_x': .5, 'center_y': .5} text: 'Item' on_release: self.set_item('New Item') ''' class Test(MDApp): def __init__(self, **kwargs): super().__init__(**kwargs) self.screen = Builder.load_string(KV) def build(self): return self.screen Test().run()
ID:(13927, 0)
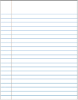
MDChip
Description 
MDChip
MDChip format:
MDChip: text: 'Check with icon' icon: 'city' check: True
No special library, no append of widget.
Example in code
from kivy.lang import Builder from kivymd.app import MDApp KV = ''' BoxLayout: MDChip: text: 'Earth' icon: 'earth' check: True selected_chip_color: .21176470535294, .098039627451, 1, 1 callback: app.callback ''' class MainApp(MDApp): def build(self): return Builder.load_string(KV) def callback(self, instance, value): pass MainApp().run()
ID:(13952, 0)
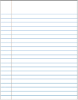
MDChip - Alternatives
Description 
MDChip
MDChip format:
MDChooseChip: MDChip: text: 'Earth' icon: 'earth' selected_chip_color: .21176470535294, .098039627451, 1, 1 MDChip: text: 'Face' icon: 'face' selected_chip_color: .21176470535294, .098039627451, 1, 1 MDChip: text: 'Facebook' icon: 'facebook' selected_chip_color: .21176470535294, .098039627451, 1, 1
No special library, no append of widget.
Example in code
from kivy.lang import Builder from kivymd.app import MDApp KV = ''' ScrollView: MDChooseChip: MDChip: text: 'Earth' icon: 'earth' selected_chip_color: .21176470535294, .098039627451, 1, 1 MDChip: text: 'Face' icon: 'face' selected_chip_color: .21176470535294, .098039627451, 1, 1 MDChip: text: 'Facebook' icon: 'facebook' selected_chip_color: .21176470535294, .098039627451, 1, 1 ''' class MainApp(MDApp): def build(self): return Builder.load_string(KV) MainApp().run()
ID:(13953, 0)
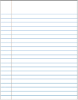
MDDatePicker
Description 
MDDatePicker
MDDatePicker format:
MDRaisedButton: text: 'Open time picker' pos_hint: {'center_x': .5, 'center_y': .5} on_release: app.show_date_picker()
Special library:
- from kivymd.uix.picker import MDDatePicker
Example in code
from kivy.lang import Builder from kivymd.app import MDApp from kivymd.uix.picker import MDDatePicker KV = ''' MDFloatLayout: MDToolbar: title: 'MDDatePicker' pos_hint: {'top': 1} elevation: 10 MDRaisedButton: text: 'Open time picker' pos_hint: {'center_x': .5, 'center_y': .5} on_release: app.show_date_picker() ''' class MainApp(MDApp): def build(self): return Builder.load_string(KV) def on_save(self, instance, value, date_range): ''' Events called when the 'OK' dialog box button is clicked. :type instance:; :param value: selected date; :type value: ; :param date_range: list of 'datetime.date' objects in the selected range; :type date_range: ; ''' print(instance, value, date_range) def on_cancel(self, instance, value): '''Events called when the 'CANCEL' dialog box button is clicked.''' def show_date_picker(self): date_dialog = MDDatePicker() date_dialog.bind(on_save=self.on_save, on_cancel=self.on_cancel) date_dialog.open() MainApp().run()
ID:(13959, 0)
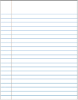
MDDatePicker, Range
Description 
MDDatePicker, Range
MDDatePicker format:
MDRaisedButton: text: 'Open time picker' pos_hint: {'center_x': .5, 'center_y': .5} on_release: app.show_date_picker()
Special library:
- from kivymd.uix.picker import MDDatePicker
Example in code
from kivy.lang import Builder from kivymd.app import MDApp from kivymd.uix.picker import MDDatePicker KV = ''' MDFloatLayout: MDToolbar: title: 'MDDatePicker' pos_hint: {'top': 1} elevation: 10 MDRaisedButton: text: 'Open time picker' pos_hint: {'center_x': .5, 'center_y': .5} on_release: app.show_date_picker() ''' class MainApp(MDApp): def build(self): return Builder.load_string(KV) def on_save(self, instance, value, date_range): ''' Events called when the 'OK' dialog box button is clicked. :type instance:; :param value: selected date; :type value: ; :param date_range: list of 'datetime.date' objects in the selected range; :type date_range: ; ''' print(instance, value, date_range) def on_cancel(self, instance, value): '''Events called when the 'CANCEL' dialog box button is clicked.''' def show_date_picker(self): date_dialog = MDDatePicker( min_date=datetime.date(2021, 2, 15), max_date=datetime.date(2021, 3, 27), ) date_dialog.open() MainApp().run()
ID:(13960, 0)
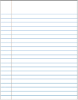
MDTimePicker
Description 
MDTimePicker
MDTimePicker format:
MDRaisedButton: text: 'Open time picker' pos_hint: {'center_x': .5, 'center_y': .5} on_release: app.show_date_picker()
Special library:
- from kivymd.uix.picker import MDTimePicker
Example in code
from kivy.lang import Builder from kivymd.app import MDApp from kivymd.uix.picker import MDTimePicker KV = ''' MDFloatLayout: MDRaisedButton: text: 'Open time picker' pos_hint: {'center_x': .5, 'center_y': .5} on_release: app.show_time_picker() ''' class MainApp(MDApp): def build(self): return Builder.load_string(KV) def show_time_picker(self): '''Open time picker dialog.''' time_dialog = MDTimePicker() time_dialog.open() MainApp().run()
ID:(13961, 0)
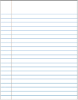
MDFileManager
Description 
MDFileManager
Example:
from kivy.core.window import Window from kivy.lang import Builder from kivymd.app import MDApp from kivymd.uix.filemanager import MDFileManager from kivymd.toast import toast KV = ''' BoxLayout: orientation: 'vertical' MDToolbar: title: 'MDFileManager' left_action_items: [['menu', lambda x: None]] elevation: 10 BoxLayout: orientation: 'vertical' spacing: 10 padding:20 MDRoundFlatIconButton: text: 'Get Url' icon: 'folder' pos_hint: {'center_x': .5, 'center_y': .6} on_release: app.file_manager_open(my_custom_label1) MDLabel: id: my_custom_label1 text: 'URL' halign: 'center' ''' class MainApp(MDApp): def __init__(self, **kwargs): super().__init__(**kwargs) Window.bind(on_keyboard=self.events) self.manager_open = False self.file_manager = MDFileManager( exit_manager=self.exit_manager, select_path=self.select_path, #previous=True, ) self.file_manager.ext = ['.xlsx', '.kv'] def build(self): return Builder.load_string(KV) def file_manager_open(self, label_id): self.file_manager.show('/') # output manager to the screen self.manager_open = True self.label_id = label_id def select_path(self, path): '''It will be called when you click on the file name or the catalog selection button. :type path: str; :param path: path to the selected directory or file; ''' self.label_id.text = path self.exit_manager() toast(path) #self.root.ids.my_custom_label1.text = path def exit_manager(self, *args): '''Called when the user reaches the root of the directory tree.''' self.manager_open = False self.file_manager.close() def events(self, instance, keyboard, keycode, text, modifiers): '''Called when buttons are pressed on the mobile device.''' if keyboard in (1001, 27): if self.manager_open: self.file_manager.back() return True MainApp().run()
ID:(13933, 0)