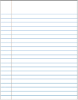
Regresión Lineal
Descripción 
plt.plot(x,y,'ro')
plt.axis([0,6,0,20])
plt.plot(np.unique(x),np.poly1d(np.polyfit(x,y,1))(np.unique(x)))
plt.show()
ID:(10380, 0)

Regresión Polinomial
Concepto 
```
const a = tf.variable(tf.scalar(Math.random()));
const b = tf.variable(tf.scalar(Math.random()));
const c = tf.variable(tf.scalar(Math.random()));
const d = tf.variable(tf.scalar(Math.random()));
```
```
const numIterations = 75;
const learningRate = 0.5;
const optimizer = tf.train.sgd(learningRate);
```
```
function predict(x) {
// y = a * x ^ 3 + b * x ^ 2 + c * x + d
return tf.tidy(() => {
return a.mul(x.pow(tf.scalar(3, 'int32')))
.add(b.mul(x.square()))
.add(c.mul(x))
.add(d);
});
}
```
```
function loss(prediction, labels) {
// Having a good error function is key for training a machine learning model
const error = prediction.sub(labels).square().mean();
return error;
}
```
```
async function train(xs, ys, numIterations) {
for (let iter = 0; iter < numIterations; iter++) {
// optimizer.minimize is where the training happens.
// The function it takes must return a numerical estimate (i.e. loss)
// of how well we are doing using the current state of
// the variables we created at the start.
// This optimizer does the 'backward' step of our training process
// updating variables defined previously in order to minimize the
// loss.
optimizer.minimize(() => {
// Feed the examples into the model
const pred = predict(xs);
return loss(pred, ys);
});
// Use tf.nextFrame to not block the browser.
await tf.nextFrame();
}
}
```
```
async function learnCoefficients() {
const trueCoefficients = {a: -.8, b: -.2, c: .9, d: .5};
const trainingData = generateData(100, trueCoefficients);
// Plot original data
renderCoefficients('#data .coeff', trueCoefficients);
await plotData('#data .plot', trainingData.xs, trainingData.ys)
// See what the predictions look like with random coefficients
renderCoefficients('#random .coeff', {
a: a.dataSync()[0],
b: b.dataSync()[0],
c: c.dataSync()[0],
d: d.dataSync()[0],
});
const predictionsBefore = predict(trainingData.xs);
await plotDataAndPredictions(
'#random .plot', trainingData.xs, trainingData.ys, predictionsBefore);
// Train the model!
await train(trainingData.xs, trainingData.ys, numIterations);
// See what the final results predictions are after training.
renderCoefficients('#trained .coeff', {
a: a.dataSync()[0],
b: b.dataSync()[0],
c: c.dataSync()[0],
d: d.dataSync()[0],
});
const predictionsAfter = predict(trainingData.xs);
await plotDataAndPredictions(
'#trained .plot', trainingData.xs, trainingData.ys, predictionsAfter);
predictionsBefore.dispose();
predictionsAfter.dispose();
}
```
```
learnCoefficients();
```
ID:(10376, 0)