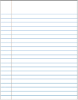
Generate directory array
Description 
If you are looking for a list of names of the directories
that are associated with the directory sample_directory
example: 'F:/go/face_scrapper/faces/samples/'
you can use the listdir command from the library < b>os:
import os # definiciones sample_directory = 'F:/go/face_scrapper/faces/samples/' # obtener arreglo de directorios classes = [] maincls = os.listdir(sample_directory) for cls in maincls: # generar list de archivos file = os.listdir(sample_directory+'/'+cls+'/') # agregar subdirectorios si contienen imagenes if len(file) > 0: classes.append(cls) # mostrar classes print(classes)

Only include folders with images.
ID:(13748, 0)
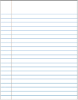
Generate directory array with subdirectories
Description 
If you are looking for a list of names of the subdirectories
that are associated with a main directory sample_directory
example: 'F:/go/face_scrapper/faces/samples/'
you can use the command listdir from the library os:
import os # definitions sample_directory = 'F:/go/face_scrapper/faces/samples/' # get directory array classes = [] maincls = os.listdir(sample_directory) for subcls in maincls: # get subdirectory array clslst = os.listdir(sample_directory+subcls+'/') for cls in clslst: file = os.listdir(sample_directory+subcls+'/'+cls+'/') # add subdirectories to the array if len(file) > 0: classes.append(cls) # show classes print(classes)
ID:(13781, 0)
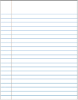
Check that there are no doubles in the arrangement
Description 
To ensure that the classes array does not contain class for each type, all elements must be verified to be unique:
import collections print([item for item, count in collections.Counter(classes).items() if count > 1]) print('number of elements:'+str(len(classes)))

The class array must not contain doubles.
ID:(13782, 0)
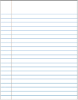
Create directories for model
Description 
To run the models, the directories are created
- train
- validate
- test
with the subdirectories of each image of the same classification depending from the work directory work_directory
example: 'F:/go/face_scrapper/faces/work/'
import os # define directories work_directory = 'F:/go/face_scrapper/faces/work/' train_path = work_directory+'train/' validate_path = work_directory+'validate/' test_path = work_directory+'test/' # create subdirectories for subcls in classes: # define train directory FOLDER_EXIST = os.path.isdir(train_path+subcls) # check and create if not FOLDER_EXIST: os.mkdir(train_path+subcls) # define validate directory FOLDER_EXIST = os.path.isdir(validate_path+subcls) # check and create if not FOLDER_EXIST: os.mkdir(validate_path+subcls) # define test directory FOLDER_EXIST = os.path.isdir(test_path+subcls) # check and create if not FOLDER_EXIST: os.mkdir(test_path+subcls)
ID:(13749, 0)
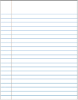
Copy images to directories
Description 
Once the directories are generated, the images must be copied to each subdirectory. To do this, you have to go through the subdirectories that depend on the sample directory sample_directory
example 'F:/go/face_scrapper/faces/samples/'
and copy them to the working directories work_directory
example 'F:/go/face_scrapper/faces/work/'
in its versions train (train), validate (validate) and test (test).
The number to be copied must have the fractions
- 80% train
- 15% validate
- 5% test
import shutil # definitions sample_directory = 'F:/go/face_scrapper/faces/samples/' work_directory = 'F:/go/face_scrapper/faces/work/' train_path = work_directory+'train/' validate_path = work_directory+'validate/' test_path = work_directory+'test/' for subcls in classes: # get subclasses subclslst = os.listdir(sample_directory+subcls) # numbers nlen = len(subclslst) if nlen > 0: nlen1 = round(nlen*0.8) if nlen1 == 0: nlen1 = 1 nlen2 = round(nlen*0.15) if nlen2 == 0: nlen2 = 1 nlen3 = round(nlen*0.05) if nlen3 == 0: nlen3 = 1 if nlen1 + nlen2 + nlen3 > nlen: npos3 = nlen - 1 else: npos3 = nlen1 + nlen2 if nlen1 + nlen2 > nlen: npos1 = nlen - 1 npos2 = nlen else: npos1 = nlen1 npos2 = nlen1 + nlen2 print(cls+','+str(nlen1)+','+str(nlen2)+','+str(nlen3)) # copy files for i in range(0,nlen1): shutil.copyfile(sample_directory+subcls+'/'+subclslst[i],train_path+subcls+'/'+subclslst[i]) for i in range(npos1,npos2): shutil.copyfile(sample_directory+subcls+'/'+subclslst[i],validate_path+subcls+'/'+subclslst[i]) for i in range(npos3,nlen): shutil.copyfile(sample_directory+subcls+'/'+subclslst[i],test_path+subcls+'/'+subclslst[i])
ID:(13750, 0)
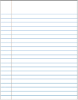
Copy images to directories with subdirectories
Description 
Once the directories are generated, the images must be copied to each subdirectory. To do this, you have to go through the subdirectories that depend on the sample directory sample_directory
example 'F:/go/face_scrapper/faces/samples/'
and copy them to the working directories work_directory
example 'F:/go/face_scrapper/faces/work/'
in its versions train (train), validate (validate) and test (test).
The number to be copied must have the fractions
- 80% train
- 15% validate
- 5% test
import shutil # definitions sample_directory = 'F:/go/face_scrapper/faces/samples/' work_directory = 'F:/go/face_scrapper/faces/work/' train_path = work_directory+'train/' validate_path = work_directory+'validate/' test_path = work_directory+'test/' # get directory array maincls = os.listdir(sample_directory) for subcls in maincls: # get subclasses temp = os.listdir(sample_directory+subcls+'/') for cls in temp: # file list filelst = os.listdir(sample_directory+subcls+'/'+cls+'/') # numbers nlen = len(filelst) if nlen > 0: nlen1 = round(nlen*0.8) if nlen1 == 0: nlen1 = 1 nlen2 = round(nlen*0.15) if nlen2 == 0: nlen2 = 1 nlen3 = round(nlen*0.05) if nlen3 == 0: nlen3 = 1 if nlen1 + nlen2 + nlen3 > nlen: npos3 = nlen - 1 else: npos3 = nlen1 + nlen2 if nlen1 + nlen2 > nlen: npos1 = nlen - 1 npos2 = nlen else: npos1 = nlen1 npos2 = nlen1 + nlen2 print(cls+','+str(nlen1)+','+str(nlen2)+','+str(nlen3)) # copy files for i in range(0,nlen1): shutil.copyfile(sample_directory+subcls+'/'+cls+'/'+filelst[i],train_path+cls+'/'+filelst[i]) for i in range(npos1,npos2): shutil.copyfile(sample_directory+subcls+'/'+cls+'/'+filelst[i],validate_path+cls+'/'+filelst[i]) for i in range(npos3,nlen): shutil.copyfile(sample_directory+subcls+'/'+cls+'/'+filelst[i],test_path+cls+'/'+filelst[i])
ID:(13783, 0)
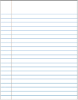
Generate images for learning process
Description 
In order to train the model, a pre-processing is carried out in which
- the images are scaled to the defined size (it is scaled regardless of whether the original image is larger, smaller and / or not square).
- contrast is increased by working with a different color palette
import numpy as np import tensorflow as tf from tensorflow import keras from tensorflow.keras.preprocessing.image import ImageDataGenerator # definitions work_directory = 'F:/go/face_scrapper/faces/work/' train_path = work_directory+'train/' validate_path = work_directory+'validate/' test_path = work_directory+'test/' # generate images train_batches = ImageDataGenerator(preprocessing_function=tf.keras.applications.vgg16.preprocess_input).flow_from_directory(directory=train_path, target_size=(224,224), classes=classes, batch_size=10) valid_batches = ImageDataGenerator(preprocessing_function=tf.keras.applications.vgg16.preprocess_input).flow_from_directory(directory=valid_path, target_size=(224,224), classes=classes, batch_size=10) test_batches = ImageDataGenerator(preprocessing_function=tf.keras.applications.vgg16.preprocess_input).flow_from_directory(directory=test_path, target_size=(224,224), classes=classes, batch_size=10, shuffle=False)

If you train your own model, the size of the images (target_size) can assume any value. However, if a pre-trained model is used, the size must be adjusted to the images of the original model.
ID:(13751, 0)
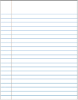
Dataformat for train, validate y test
Description 
La función ImageDataGenerator genera archivos con el formato dado por:
{'image_data_generator':
'target_size': (224, 224),
'color_mode': 'rgb',
'data_format': 'channels_last',
'image_shape': (224, 224, 3),
'save_to_dir': None,
'save_prefix': '',
'save_format': 'png',
'interpolation': 'nearest',
'split': None,
'subset': None,
'directory': 'F:/go/face_scrapper/faces/work/test/',
'classes': array([ 0, 1, 2, ..., 288, 289]),
'class_mode': 'categorical',
'dtype': 'float32',
'samples': 663,
'num_classes': 290,
'class_indices': {'arraya-andi': 0, 'arraya-marielle': 1, ...,'wagner-mancho': 288, 'wagner-richard': 289},
'filenames': ['arraya-andi
eg087_002_003_603-625-293-375.jpg',
...,
'wagner-richard\\sony-cybershot-20011204-1806002_001_201-163-141-172.jpg'],
'_filepaths': ['F:/go/face_scrapper/faces/work/test/arraya-andi
eg087_002_003_603-625-293-375.jpg',
...,
'F:/go/face_scrapper/faces/work/test/wagner-richard\\sony-cybershot-20011204-1806002_001_201-163-141-172.jpg'],
'n': 663,
'batch_size': 10,
'seed': None,
'shuffle': False,
'batch_index': 4,
'total_batches_seen': 140,
'lock':
'index_array': array([ 0, 1, 2, ..., 661, 662]),
'index_generator':
test_
ID:(13799, 0)